vRealize Orchestrator Configuration Elements in a nutshell
When using an older vRO workflows (vRealize Orchestrator) I stumbled upon the problem that too many information needed to be entered manually. In this case it is easy to enter information incorrectly so I was looking for a possibility to avoid unnecessary inputs. During my researches I stumbled upon Configuration Elements. Reading two blog posts (here and here) I focussed on this and implemented appropriate functions in my home lab. This blog post describes the whole procedure a little bit more detailed than the sources mentioned above.
But... why?
Provisioning virtual machines is a real-world example. During this, various parameters need to be set - e.g.:
- IP address
- Network mask
- Gateway
- DNS server and search domains
If you add also those parameters in the presentation layer of a workflow, the user experience will get confusing and also errors will be more likely if you need to enter information manually. It would be more handy to store these information in a configuration file. In this case, selecting a template will be sufficient to apply all the settings - user experience will also benefit from this:
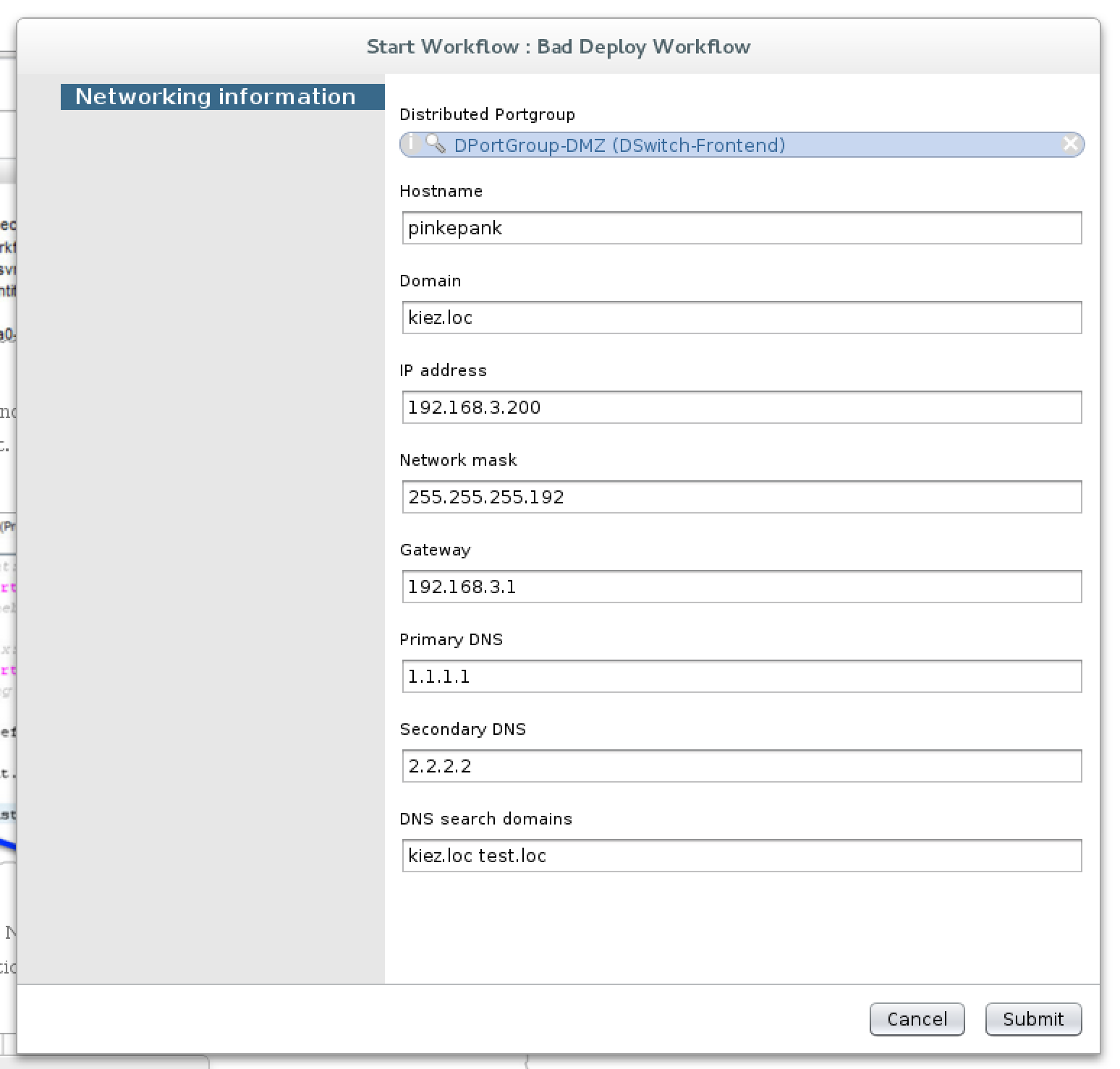
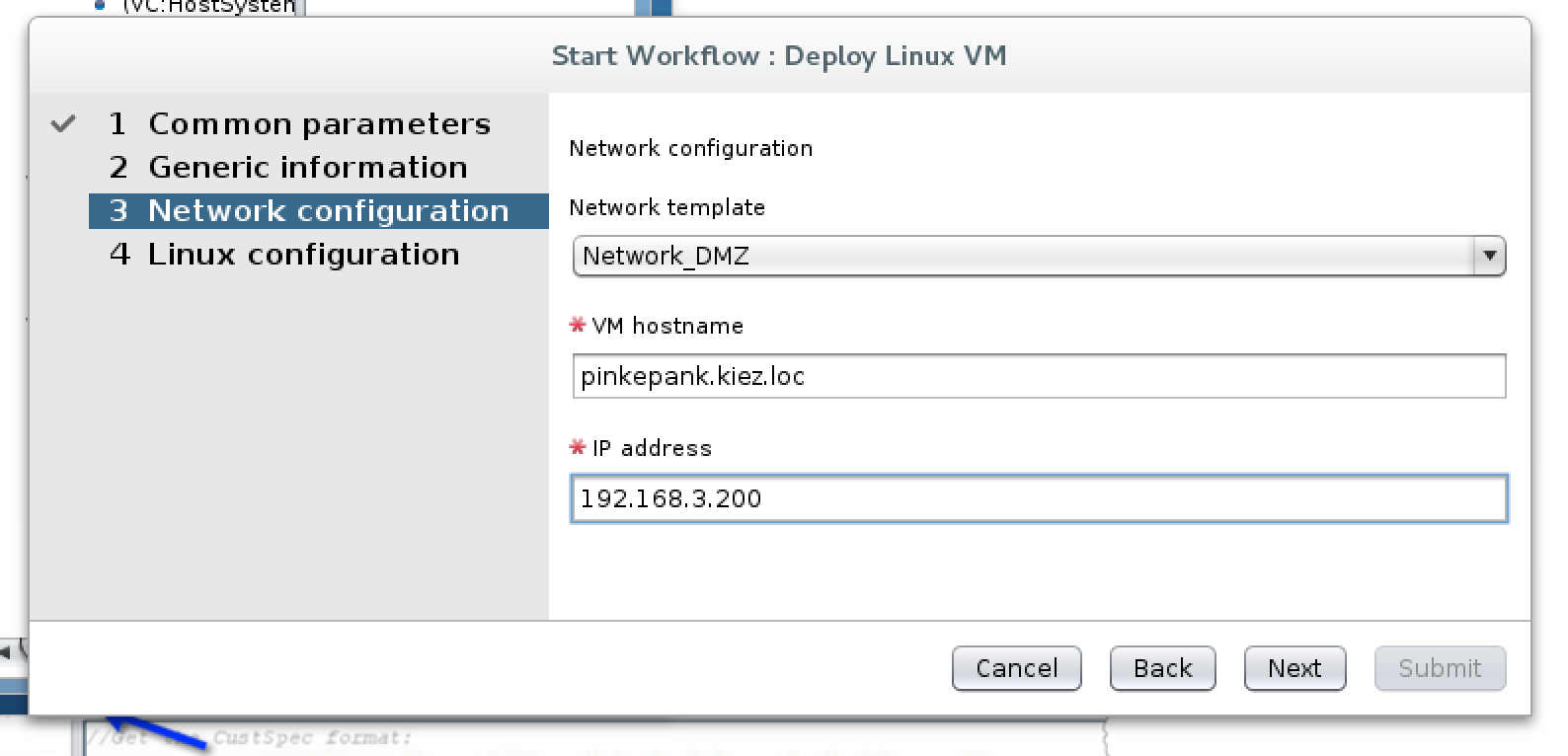
Procedure
First of all, network configurations need to be stored within vRO. It is recommended to create a dedicated configuration folder in the Configuration tab. I created a dedicated configuration file per network and assigned the required attributes:
I stored my network configurations in another sub-folder NetworkConfigs
as I'm looking forward to create additional configurations in the future.
Afterwards, two "code snippets", actions, are required to implement the following logic:
getConfigurationElements
- reading all configuration file names within a foldergetConfigurationElementValue
- retrieving a setting from a configuration file
The first function is used in the presentation layer of a workflow to make the configuration files selectable from a dropdown box (see also the second screenshot above). The second function can be used later in a workflow to retrieve the particular settings and use them - e.g. to assign network information to a cloned VM.
The first module
Before the particular actions are created, a module needs to be created. There is a convention for naming modules - it looks like this:
1tld.company.name
I have chosen com.stdevel.generic
for my module - generic because it contains very basic functions that could be used in a wide range of workflows. If you would plan to create additional actions for e.g. monitoring it would be a great idea to create another module for this - e.g. com.stdevel.monitoring
. To create a module, the Design pane (gear wheel icon) needs to be selected. Clicking New module will open a window for entering the name.
getConfigurationElements
For easier reading, I will name Configuration Elements as configuration files in the following sections of this post.
In the new module, click Add action to create an action.
The first action, getConfigurationElements
, will execute the following:
- Reading the configuration category from the submitted path (Stankowic/NetworkConfigs in the example above)
- Retrieving all configuration files within a category or aborting if the path is invalid
- Reading all configuration file names and storing them in an array
- Returning the array
The source code looks like this:
1//get category
2var category = Server.getConfigurationElementCategoryWithPath(categoryPath);
3
4//die in a fire if non-existent
5if (category == null) {
6 throw "Configuration element category '" + categoryPath + "' not found or empty!";
7}
8
9//get _all_ the elements
10var elements = category.configurationElements;
11var result = [];
12
13//retrieve names
14for (i = 0; i < elements.length; i++) {
15 System.log("Found configuration element '" + elements[i].name + "'");
16 result.push(elements[i].name);
17}
18
19//return results
20return result
By the way, the action source code is available on GitHub: [click me!]
To test this action, I created a workflow which prompts a path and lists all configuration files in the console:
This workflow can be downloaded on GitHub: [click me!]
getConfigurationElementValue
Listing all configuration files works - the next step is to retrieve a setting from a particular configuration file. The next action will do the following:
- Reading the configuration category from the submitted path
- Reading configuration files, selecting by the specified name
- Reading the settings, selecting the value based on the specified setting name
- Returning the value
The source code looks like this:
1//get category
2var category = Server.getConfigurationElementCategoryWithPath(categoryPath);
3
4//die in a fire if non-existent
5if (category == null) {
6 throw "Configuration element category '" + categoryPath + "' not found or empty!";
7}
8
9//get _all_ the elements
10var elements = category.configurationElements;
11var result = [];
12
13//retrieve names
14for (i = 0; i < elements.length; i++) {
15 if (elements[i].name == elementName) {
16 //found required element
17 var attribute = elements[i].getAttributeWithKey(attributeName);
18 if (attribute != null) {
19 System.log("Found attribute '" + attributeName + "' in '" + elementName + "' with value '" + attribute.value + "'");
20 return attribute.value;
21 }
22 else {
23 throw "Attribute '" + attributeName + "' not found!";
24 }
25 }
26}
This action's source code is also available on GitHub: [click me!]
For testing purposes it is a good idea to create another simple workflow which prompts for a configuration path, configuration file and setting before printing the value on the console:
This workflow is also available on GitHub: [click me!]
Conclusion
Like mentioned in the beginning of this post, provisioning virtual machines is an use-case for the implemented actions. In a future blog post I will focus on this. 🙂